Clue
This guide shows how you can program the Adafruit Clue to function as a remote control for the Shared Game Timer, and how to 3D print an enclosure for it and its battery to make it nice and portable.
Overview
The Shared Game Timer has a open-ended bluetooth module that allow you to write to and read from Bluetooth Low Energy (LE) devices. These can be things like LED lights, buttons etc. In this guide, we will program the Adafruit Clue board to be a really cool little remote control.
Adafruit is an awesome electronics company based in New York but they sell world-wide. Their mission is to make electronics approachable and fun, and one of their entry-level boards is the Clue. It has LCD screen, two physical buttons, three capacitive touch buttons and much more.
The screen is the big selling point. The other guides use LEDs to show the current player color, but you cannot show Black with a light! The LCD screen has no such issue.
You can power the board from a standard USB power pack, but to make this remote control easier to pass around a board game table, we will also 3D print a small enclosure for the battery and a power switch.
Components
The main component is of course the Clue board, and, if you will power it via USB, that is all you need. (and a USB power pack and a USB micro cable of course)
But, if you want to go portable, also get the LiPo battery and a charger. Both the 400mA and 500mA batteries fit, and you only need one. The other bits are for the enclosure power switch.
The Enclosure
To make the board easy to pass around the gaming table, you want the battery and the board to be one unit.
You could go the McGyver route and simply tape them together with electrical tape... But, for a more graceful solution, 3D print a battery enclosure.
Assuming you don't have a 3D printer, maybe you can find one at your school, university or there may be a local company that allow you to print items? Failing that, there are many online companies that will happily print and send you items.
The 3D print files are located at this Thingiverse page. To assemble the power switch and the case in general, follow this guide, which is for a slightly different case. Ignore the watch strap parts.
I made some minor tweaks to the guide.
- The back plate was a bit loose, so I put a piece of electrical tape on the inside of back-plate wall to make the fit tighter.
- I taped the battery to the back plate to make it not tumble around inside the enclosure.
- I used the 400 mA battery, but I have also tried it with the 500 mA battery. It is a tighter fit, but works, and would give you more juice, so I suggest you use that.
- The LCD frame I didn't bother putting on. I think it is only for looks.
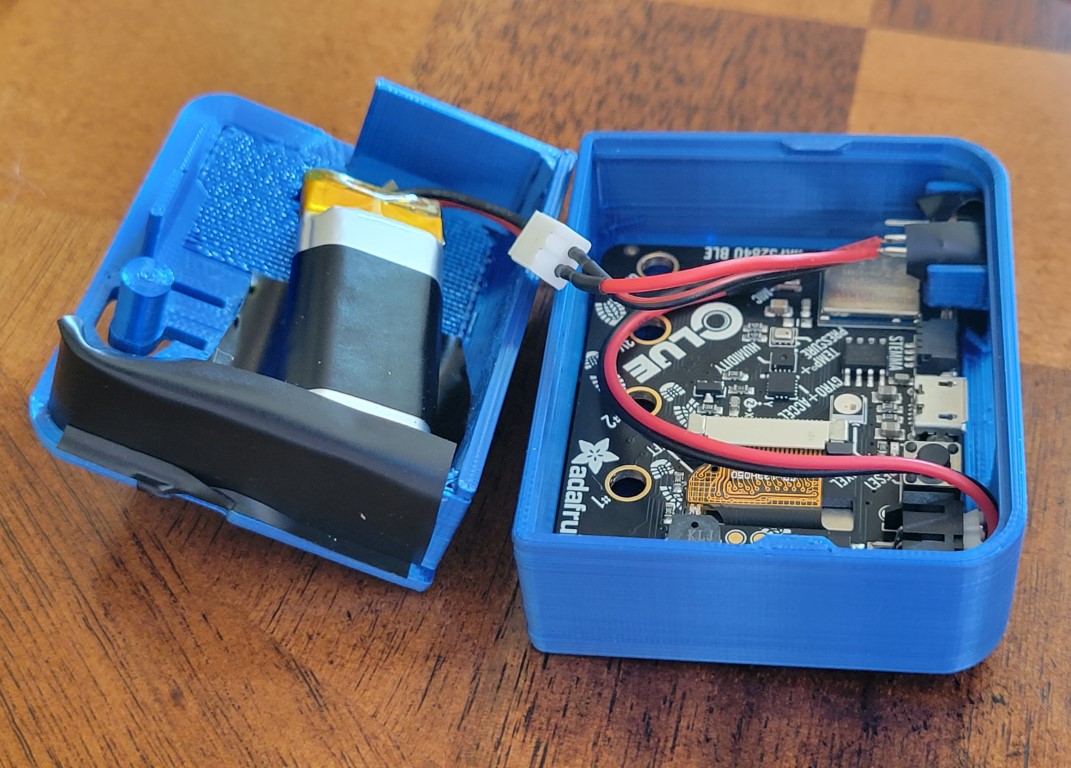
Programming the Clue
We will be programming the Clue using Circuit Python. There is a bit of setup to make this all work. I will assume that you know nothing about programming, and that your board is brand new.
- Install Circuit Python on your board by following this setup guide page. You should now have a disk drive called
CIRCUITPY
. - Follow this guide to install some basic software libraries. Take all the libraries that the guide tells you to install, but you will also need a few extra. In the end, you should have these installed under
/lib
: adafruit_display_shapes, adafruit_display_text, adafruit_ble, adafruit_bitmap_font, adafruit_apds9960, adafruit_register, adafruit_lsm6ds, adafruit_clue.mpy, neopixel.mpy, adafruit_bmp280.mpy, adafruit_lis3mdl.mpy, adafruit_sht31d.mpy. If you struggle at this stage, check out this page for more details about installing libraries. - Download and unzip ClueCircuitPythonFiles.zip to your Clue device, overwriting the code.py file (safe-copy it if you like).
- The top of the
code.py
file has some variables that you can edit to customize how the remote works. You will find them between the comments saying '# Constants and Setup' and 'END OF THINGS YOU SHOULD MESS WITH'. Go ahead and play around with the variables. You can probably do this editing in any text editor, but I recommend you install the Mu Editor. See these pages for help on editing code: Installing the Mu Editor, Creating and Editing Code, Connecting to the Serial Console (if you want to see debug/error messages)
If you did all of the above, you should see the SGT logo with a Bluetooth icon, meaning it is ready to be connected to via Bluetooth
Connecting it to the Shared Game Timer
The Shared Game Timer Bluetooth connectivity is quite open-ended, able to connect to many different devices. This flexibility also means that the process of setting it up can be a bit confusing, but this step-by-step guide should hopefully help, and if you struggle, feel free to email me and I will be happy to assist.
Go to any Shared Game Timer game (feel free to create a toy one just to try it out), open the menu, open the Remote Control menu and click into the Bluetooth LE page.
Click the Device Configuration link.
If you have not yet added the Nordic UART service, click the 'Add Nordic UART' button.
You should now see the Service in the list of services. Click the button and you should be asked to connect to the Bluetooth device. You should see it available named 'Clue'. Click it and then Pair.
You should now see two sections, Write Script and Action Mapping. For more information about these sections, see the the Bluetooth Remote Control Overview page for more details.
The Shared Game Timer can ask the device for its preferred Write Script and Action Mapping by clicking the 'Request Setup Suggestion' button. This should populate the two sections. You should leave the Write Script as is, as sending the wrong commands will not work. The Actions Mapping, however, is only a suggestion, and you are free to edit it as you see fit. Remember to hit the 'Save' buttons on both sections.
The suggested 'Write Script' should be this:
0 sgtTimerMode;sgtState;sgtColor;sgtTurnTime;sgtName;sgtPlayerTime;sgtTotalPlayTime%0A
What it basically says is, "0 milliseconds after triggering, send the current timer mode, game state, color, current turn time, player name, total time taken by the player and total time for all players, followed by the new line character. For example, it might send cu;pl;ff0033;15;Gustav;400;800
. Give it a name (e.g. Clue Write) and hit Save.
Now look at the Action Mapping section. In the 'Log' you will see all the events sent from the Clue to the Shared Game Timer. Try pressing the left A button. You should see 'Button A' appear. Try pressing both A and B. You should see 'Button AB'.
Now it is up to you to decide which events should correspond to which actions in the Shared Game Timer.
The following table shows all the things the Clue can do.
Event | Description |
---|---|
Button A | Left button |
Button B | Right button |
Button AB | Both buttons |
Connect | Automatic at connection |
Covered/Uncovered | Cover the light sensor above the screen. |
Gesture direction | Wave your hand across the light sensor. |
To/From orientation | Turn the Clue to or away from a certain orientation. |
Swipe LTR | Swipe across the touch pads, left-to-right. (0>1>2) |
Swipe RTL | Swipe across the touch pads, right-to-left. (2>1>0) |
Shake | When shaking the Clue. |
direction can be Up, Down, Left, Right | |
orientation can be Standing, Upside Down, Face Down, Face Up, Right, Left | |
The Covered and Gesture features are mutually exclusive. Edit the code.py file to choose which one to activate. | |
The Orientation feature can end up sending a lot of data over bluetooth. Edit the code.py file to deactivate orientations you do not need. | |
The Shake feature can be activated/deactivated in code.py . |
When defining keywords for your action map, remember that the Shared Game Timer will evaluate each of the action mappings in order and trigger the first one that contains the keyword.
So the keyword 'Swipe' will match both 'Swipe RTL' and 'Swipe LTR'.
This is also why we must put 'Button AB' above 'Button A'. As a rule, the most expressive keywords should go at the top.
We are now all done to use the remote! Go back to the Bluetooth Remote page, and you should see the Write Script and Activation Map we just created. Activate both. For the Write Script, click the 'Tick all checkboxes' button, and also tick the 'Whenever target state becomes active' as well as 'When Polled'. Finally, click Connect and you are now DONE! 🥳
Adjusting screen brightness
After a successful Bluetooth connection, you can cycle through different screen brightnesses by tapping the #2 capacitive touch pad.